Qtimer Signal Slot
- Qtimer Signal Slot Example
- Qtimer Signal Slot Games
- Qtimer Signal Slot Car
- Qtimer Signal Slot Game
- Qtimer Signal Slot Machine
The timer triggers the timeout signal when the time is over and this will be called in the main-event loop. QTimer::singleShot simple usage. The QTimer::singleShot is used to call a slot/lambda asynchronously after n ms. The basic syntax is: QTimer::singleShot(myTime, myObject, SLOT(myMethodInMyObject)). It's good practice to give a parent to your QTimer to use Qt's memory management system. Update is a QWidget function - is that what you are trying to call or not? If number 2 does not apply, make sure that the function you are trying to trigger is declared as a slot in the header. C (Cpp) QTimer::start - 30 examples found. These are the top rated real world C (Cpp) examples of QTimer::start extracted from open source projects. You can rate examples to help us improve the quality of examples. The QTimer class provides repetitive and single-shot timers. The QTimer class provides a high-level programming interface for timers. To use it, create a QTimer, connect its timeout signal to the appropriate slots, and call start. From then on it will emit the timeout signal at constant intervals.
The QTimer
class provides repetitive and single-shot timers. More…
Synopsis¶
Functions¶
def
interval
()def
isActive
()def
isSingleShot
()def
remainingTime
()def
setInterval
(msec)def
setSingleShot
(singleShot)def
setTimerType
(atype)def
timerId
()def
timerType
()
Slots¶
Static functions¶
def
singleShot
(arg__1, arg__2)def
singleShot
(msec, receiver, member)def
singleShot
(msec, timerType, receiver, member)
Detailed Description¶
The QTimer
class provides a high-level programming interface for timers. To use it, create a QTimer
, connect its timeout()
signal to the appropriate slots, and call start()
. From then on, it will emit the timeout()
signal at constant intervals.
Example for a one second (1000 millisecond) timer (from the Analog Clock example):
From then on, the update()
slot is called every second.
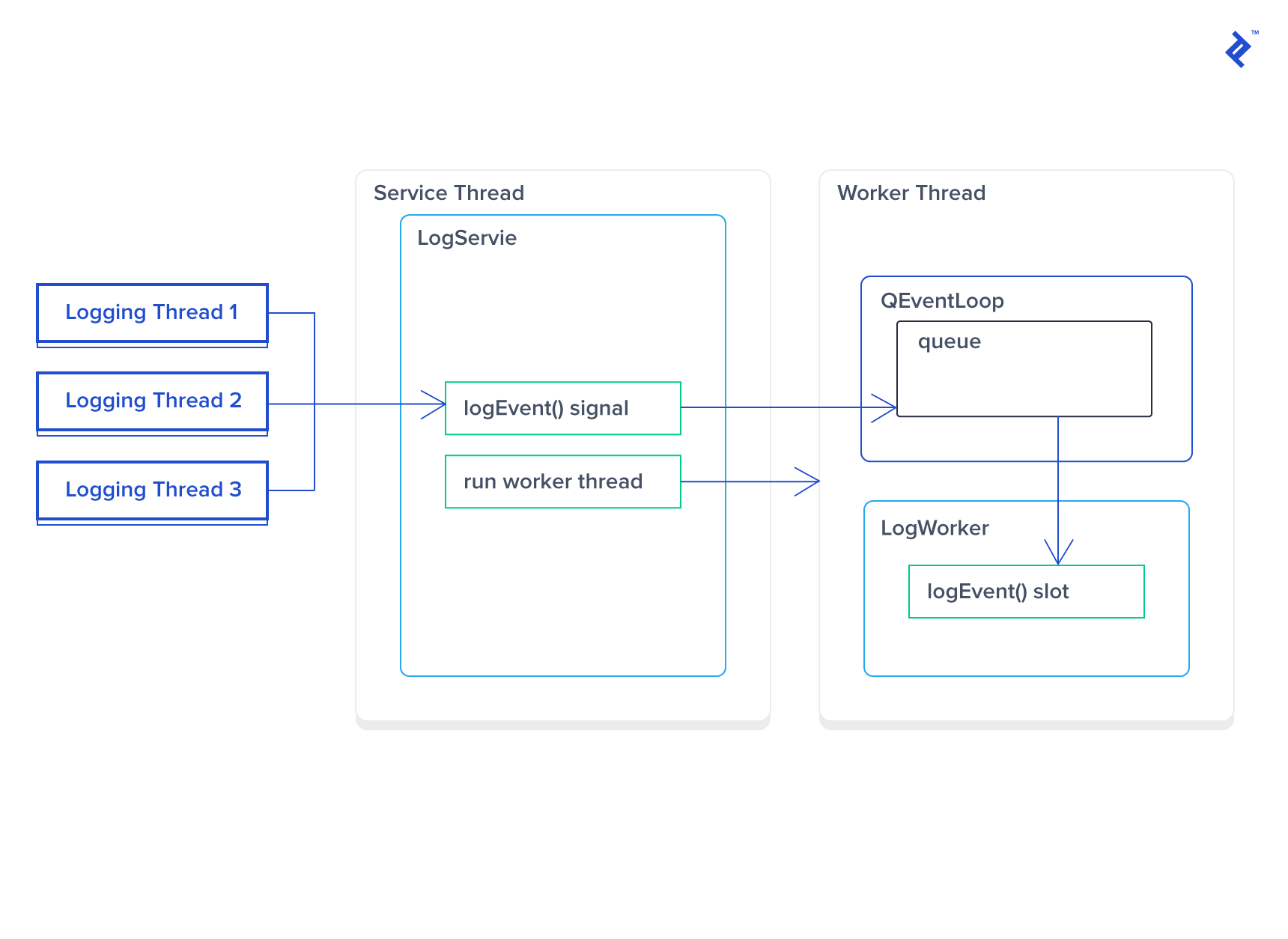
You can set a timer to time out only once by calling setSingleShot
(true). You can also use the static singleShot()
function to call a slot after a specified interval:
In multithreaded applications, you can use QTimer
in any thread that has an event loop. To start an event loop from a non-GUI thread, use exec()
. Qt uses the timer’s threadaffinity
to determine which thread will emit the timeout()
signal. Because of this, you must start and stop the timer in its thread; it is not possible to start a timer from another thread.
As a special case, a QTimer
with a timeout of 0 will time out as soon as all the events in the window system’s event queue have been processed. This can be used to do heavy work while providing a snappy user interface:
From then on, processOneThing()
will be called repeatedly. It should be written in such a way that it always returns quickly (typically after processing one data item) so that Qt can deliver events to the user interface and stop the timer as soon as it has done all its work. This is the traditional way of implementing heavy work in GUI applications, but as multithreading is nowadays becoming available on more and more platforms, we expect that zero-millisecond QTimer
objects will gradually be replaced by QThread
s.
Accuracy and Timer Resolution¶
Qtimer Signal Slot Example
The accuracy of timers depends on the underlying operating system and hardware. Most platforms support a resolution of 1 millisecond, though the accuracy of the timer will not equal this resolution in many real-world situations.
The accuracy also depends on the timertype
. For PreciseTimer
, QTimer
will try to keep the accuracy at 1 millisecond. Precise timers will also never time out earlier than expected.
For CoarseTimer
and VeryCoarseTimer
types, QTimer
may wake up earlier than expected, within the margins for those types: 5% of the interval for CoarseTimer
and 500 ms for VeryCoarseTimer
.
All timer types may time out later than expected if the system is busy or unable to provide the requested accuracy. In such a case of timeout overrun, Qt will emit timeout()
only once, even if multiple timeouts have expired, and then will resume the original interval.
Alternatives to QTimer¶
An alternative to using QTimer
is to call startTimer()
for your object and reimplement the timerEvent()
event handler in your class (which must inherit QObject
). The disadvantage is that timerEvent()
does not support such high-level features as single-shot timers or signals.
Another alternative is QBasicTimer
. It is typically less cumbersome than using startTimer()
directly. See Timers for an overview of all three approaches.
Some operating systems limit the number of timers that may be used; Qt tries to work around these limitations.
See also
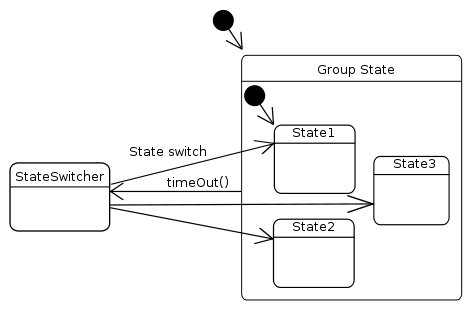
QTimer
([parent=None])¶Constructs a timer with the given parent
.
PySide2.QtCore.QTimer.
interval
()¶int
See also
PySide2.QtCore.QTimer.
isActive
()¶bool
Returns true
if the timer is running (pending); otherwise returns false.
PySide2.QtCore.QTimer.
isSingleShot
()¶bool
PySide2.QtCore.QTimer.
remainingTime
()¶int
PySide2.QtCore.QTimer.
setInterval
(msec)¶msec – int
PySide2.QtCore.QTimer.
setSingleShot
(singleShot)¶singleShot – bool
See also
PySide2.QtCore.QTimer.
setTimerType
(atype)¶atype – TimerType
PySide2.QtCore.QTimer.
singleShot
(arg__1, arg__2)¶arg__1 –
int
arg__2 –
PyCallable
PySide2.QtCore.QTimer.
singleShot
(msec, timerType, receiver, member)msec –
int
timerType –
TimerType
receiver –
QObject
member – str
This is an overloaded function.
This static function calls a slot after a given time interval.
It is very convenient to use this function because you do not need to bother with a timerEvent
or create a local QTimer
object.
The receiver
is the receiving object and the member
is the slot. The time interval is msec
milliseconds. The timerType
affects the accuracy of the timer.
Qtimer Signal Slot Games
See also
PySide2.QtCore.QTimer.
singleShot
(msec, receiver, member)msec –
int
receiver –
QObject
member – str
This static function calls a slot after a given time interval.
It is very convenient to use this function because you do not need to bother with a timerEvent
or create a local QTimer
object.
Example:
This sample program automatically terminates after 10 minutes (600,000 milliseconds).
The receiver
is the receiving object and the member
is the slot. The time interval is msec
milliseconds.
See also
PySide2.QtCore.QTimer.
start
()¶This function overloads .
Starts or restarts the timer with the timeout specified in interval
.
If the timer is already running, it will be stopped
and restarted.
If singleShot
is true, the timer will be activated only once.
PySide2.QtCore.QTimer.
start
(msec)msec – int
Starts or restarts the timer with a timeout interval of msec
milliseconds.
If the timer is already running, it will be stopped
and restarted.
If singleShot
is true, the timer will be activated only once.
PySide2.QtCore.QTimer.
stop
()¶Stops the timer.
PySide2.QtCore.QTimer.
timerId
()¶int
Returns the ID of the timer if the timer is running; otherwise returns -1.
PySide2.QtCore.QTimer.
timerType
()¶TimerType
See also
© 2018 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.
Multithreading technology is used to design three methods, one is to use counter module QTimer, the other is to use multithreading module QThread, and the other is to use event processing function.
QTimer
If you want to perform an operation periodically in the application summary, such as periodically detecting the CPU of the host, you need to use the QTimer (timer). The QTimer class provides repetitive and single timers. To use the timer, you need to first create an instance of the QTImer, connect its timeout signal to the corresponding slot, and call start.
The demo pops up a window, which disappears after 10 seconds.
QThread
QThread is the core underlying class in Qt threads
Thread instances can be invoked directly when using threads, and threads can be started by calling its start() function. After starting threads, the run method implemented by threads can be invoked automatically. This method starts with the execution function of threads.
The thread task of the business is written in the run function, and the thread ends when run exits. QThread has strarted and finished signals. It can specify slot functions for these two signals, and specify a section of code to initialize and release resources after the start and end of the thread.
Common methods
- start
- wait
- sleep
Although the interface data display and data read-write are separated, if the data read-write is very time-consuming, the interface will be stuck.
We use loops to simulate very time-consuming work. When the test button is clicked, the program interface stops responding directly. It is not updated until the end of the loop, and the timer is always displayed at 0.
All windows in PyQt are in the main thread of the UI, which blocks the UI thread by performing time-consuming operations, thus stopping the window from responding. If the window does not respond for a long time, it will affect the user experience. To avoid this problem, use the QThread to open a new thread to perform time-consuming operations on that thread.
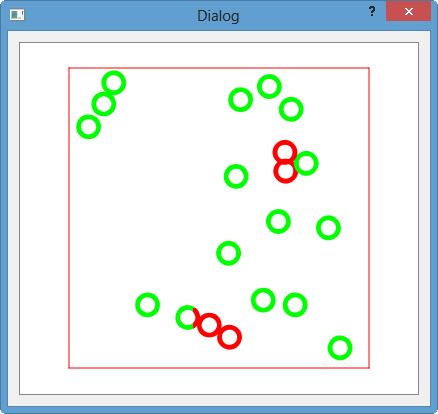
Qtimer Signal Slot Car
WorkThread inherits from the QThread class and rewrites its run function. The run() function is what the new thread needs to execute. In the run function, a loop is executed, and then the calculated signal is transmitted.
Qtimer Signal Slot Game
event processing
PyQt uses two mechanisms for event handlers: high-level signal and slot mechanisms and low-level event handlers. We introduce the use of the low-level event handler, the processEvents() function, whose function is to process time, simply to refresh the page.
For a time-consuming program, PyQt has to wait for the program to finish execution before it can proceed to the next step, which is shown as Katon on the page.
Qtimer Signal Slot Machine
Added by victor78 on Thu, 26 Sep 2019 10:33:26 +0300